The #[repr(packed)] annotation is new to us. Usually, a struct will have padding either between fields or at the end of the struct. This happens even when we’ve specified #[repr(C)].
The reason has to do with efficient access to the data stored in the struct by not having to make multiple fetches to get the data stored in a struct field. In the case of the Event struct, the usual padding would be adding 4 bytes of padding at the end of the events field. When the operating system expects a packed struct for Event, and we give it a padded one, it will write parts of event_data to the padding between the fields. When you try to read event_data later on, you’ll end up only reading the last part of event_data, which happened to overlap and get the wrong data
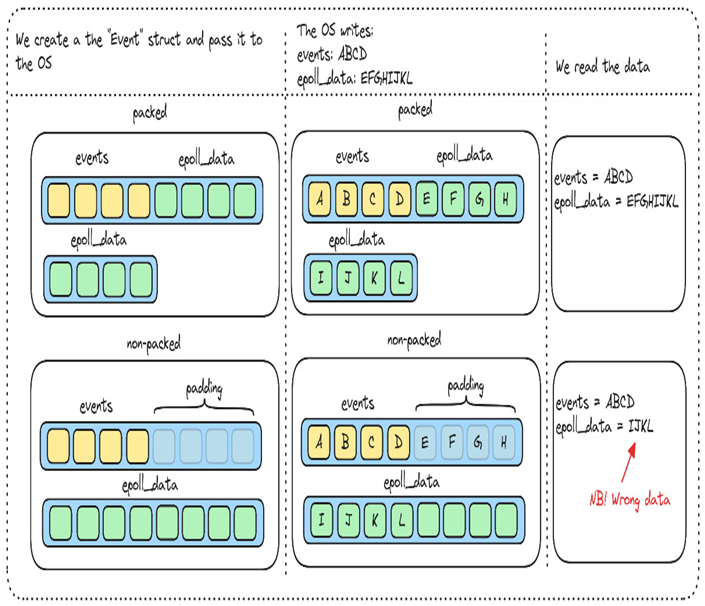
The fact that the operating systemexpects a packed Event struct isn’t obvious by reading the manpages for Linux, so you have to read the appropriate C header files to know for sure. You could of course simply rely on the libc crate (https://github.com/rust-lang/libc), which we would do too if we weren’t here to learn things like this for ourselves.
So, now that we’ve finished walking through the code, there are a few topics that we promised to get back to.
Bitflags and bitmasks
You’ll encounter this all the time when making syscalls (in fact, the concept of bitmasks is pretty common in low-level programming). A bitmask is a way to treat each bit as a switch, or a flag, to indicate that an option is either enabled or disabled.
An integer, such as i32, can be expressed as 32 bits. EPOLLIN has the hex value of 0x1 (which is simply 1 in decimal). Represented in bits, this would look like 00000000000000000000000000000001.
EPOLLET, on the other hand, has a value of 1 << 31. This simply means the bit representation of the decimal number 1, shifted 31 bits to the left. The decimal number 1 is incidentally the same as EPOLLIN, so by looking at that representation and shifting the bits 31 times to the left, we get a number with the bit representation of 10000000000000000000000000000000.
The way we use bitflags is that we use the OR operator, |, and by OR’ing the values together, we get a bitmask with each flag we OR’ed set to 1. In our example, the bitmask would look like 10000000000000000000000000000001.
The receiver of the bitmask (in this case, the operating system) can then do an opposite operation, check which flags are set, and act accordingly.
We can create a very simple example in code to show how this works in practice (you can simply run this in the Rust playground or create a new empty project for throwaway experiments such as this):
fn main() {
let bitflag_a: i32 = 1 << 31;
let bitflag_b: i32 = 0x1;
let bitmask: i32 = bitflag_a | bitflag_b;
println!(“{bitflag_a:032b}”);
println!(“{bitflag_b:032b}”);
println!(“{bitmask:032b}”);
check(bitmask);
}
fn check(bitmask: i32) {
const EPOLLIN: i32 = 0x1;
const EPOLLET: i32 = 1 << 31;
const EPOLLONESHOT: i32 = 0x40000000;
let read = bitmask & EPOLLIN != 0;
let et = bitmask & EPOLLET != 0;
let oneshot = bitmask & EPOLLONESHOT != 0;
println!(“read_event?
{read}, edge_triggered: {et}, oneshot?: {oneshot}”)
}
This code will output the following:
10000000000000000000000000000000
00000000000000000000000000000001
10000000000000000000000000000001
read_event?
true, edge_triggered: true, oneshot?: false
The next topic we will introduce in this chapter is the concept of edge-triggered events, which probably need some explanation.
Leave a Reply